This problem is from Leetcode – Detect Capital. Problem statement is as below:
We define the usage of capitals in a word to be right when one of the following cases holds:
- All letters in this word are capitals, like
"USA"
. - All letters in this word are not capitals, like
"leetcode"
. - Only the first letter in this word is capital, like
"Google"
.
Given a string word
, return true
if the usage of capitals in it is right.
Solution
To determine if the usage of capitals in a given word is correct according to the specified rules, we can implement a function that checks the following conditions:
- All letters are uppercase.
- All letters are lowercase.
- Only the first letter is uppercase, and the rest are lowercase.
See the code sample below:
public boolean detectCapitalUse(String word) {
Boolean isCapital = false;
// check if all chars are lowercase
if(word.equals(word.toLowerCase())) {
return true;
}
// check if all chars are uppercase
else if(word.equals(word.toUpperCase())) {
return true;
}
// check if first char is uppercase and rest are lowercase
if(Character.isUpperCase(word.charAt(0))) {
String str = word.substring(1, word.length());
if(str.equals(str.toLowerCase())) {
return true;
}
}
return isCapital;
}
You can checkout the code from Github here: Detect Caital – First Approach. See the performance of this approach below:
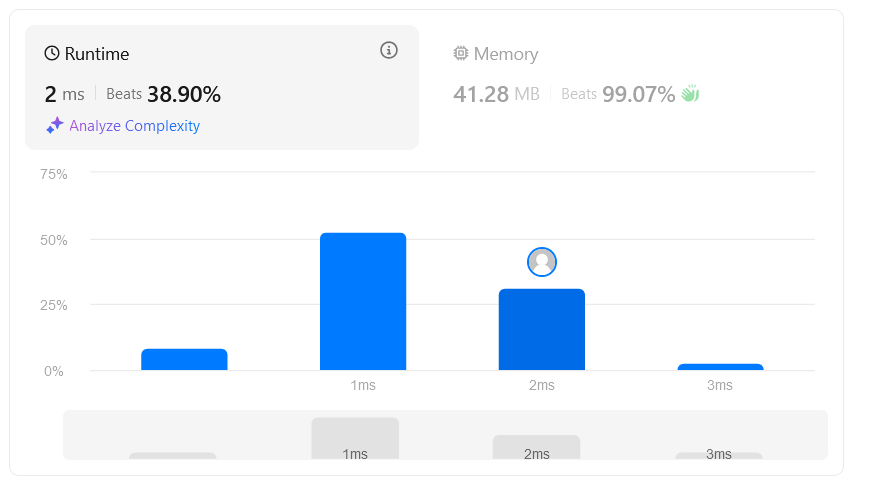