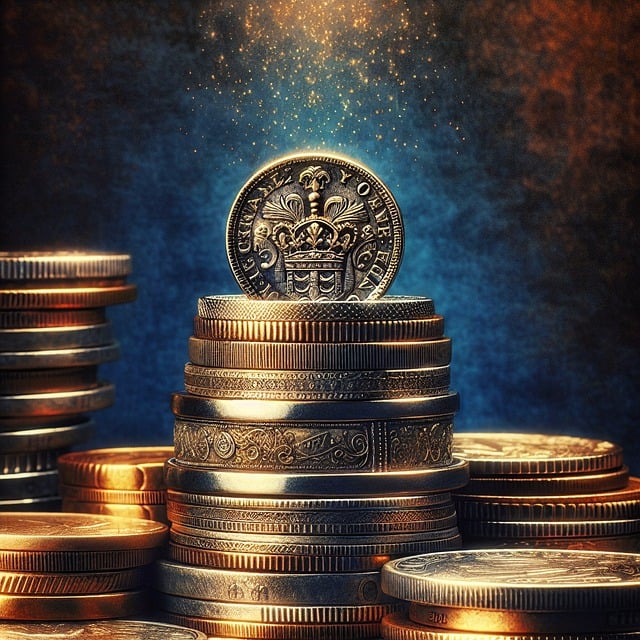
Problem Statement
This problem is from Leetcode – Arranging Coins
. The problem statement is as below:
You have n coins and you want to build a staircase with these coins. The staircase consists of k rows where the ith row has exactly i coins. The last row of the staircase may be incomplete.
Given the integer n, return the number of complete rows of the staircase you will build.
Solution
This problem is easy enough. In awhile loop simply keep subtracting the row count
from coins left
. Break out of the loop when coins left
is less than row count
. See the code sample below:
public int arrangeCoins(int n) {
// the current staircase row
int row = 0;
// coins left
int coinsLeft = n;
while(true) {
// subtract the row number from coins left
coinsLeft = coinsLeft - row;
// increment row count
row++;
// if coins left is less than row count then break out of the loop
if(coinsLeft < row) {
break;
}
}
return row-1;
}
You can checkout the code from Github here: Arranging Coins. See the performance of this approach below:
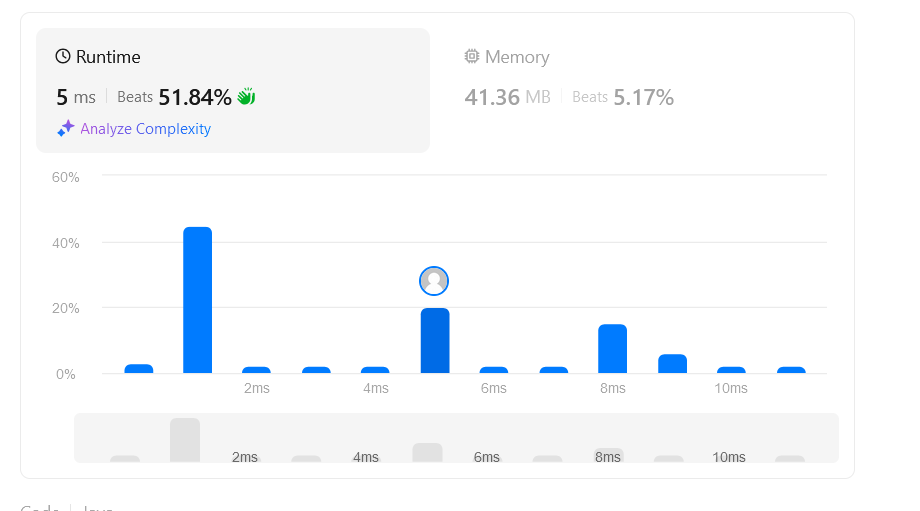
See the complexity as computed by Leetcode:
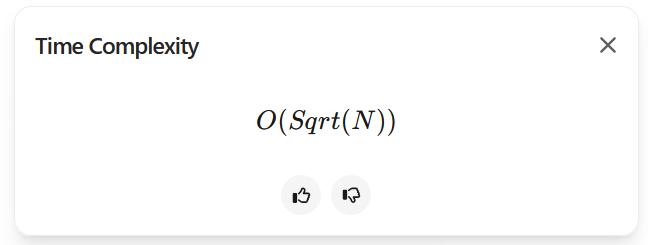