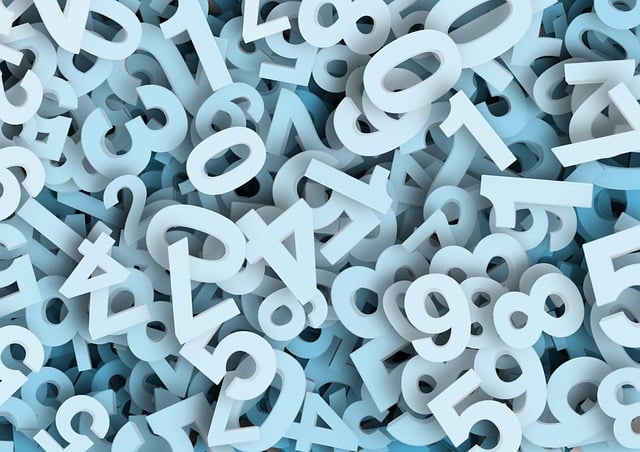
Problem Statement
This problem is from Leetcode – Number Complement
. The problem statement is as below:
The complement of an integer is the integer you get when you flip all the 0’s to 1’s and all the 1’s to 0’s in its binary representation.
- For example, The integer 5 is “101” in binary and its complement is “010” which is the integer 2.
Given an integer num, return its complement.
Solution
This problem is simple enough. We first compute the binary of the given decimal and then get its complement. See the code sample below:
public int findComplement(int num) {
// compute binary of given decimal
String bin = Integer.toBinaryString(num);
StringBuilder compStr = new StringBuilder();
// compute complement of this binary
for(int i=0; i<bin.length(); i++) {
if(bin.charAt(i) == '0') {
compStr = compStr.append('1');
}
if(bin.charAt(i) == '1') {
compStr = compStr.append('0');
}
}
// return corresponding decimal
return Integer.parseInt(compStr.toString(),2);
}
You can checkout the code from Github here: Number Complement. See the performance of this approach below:
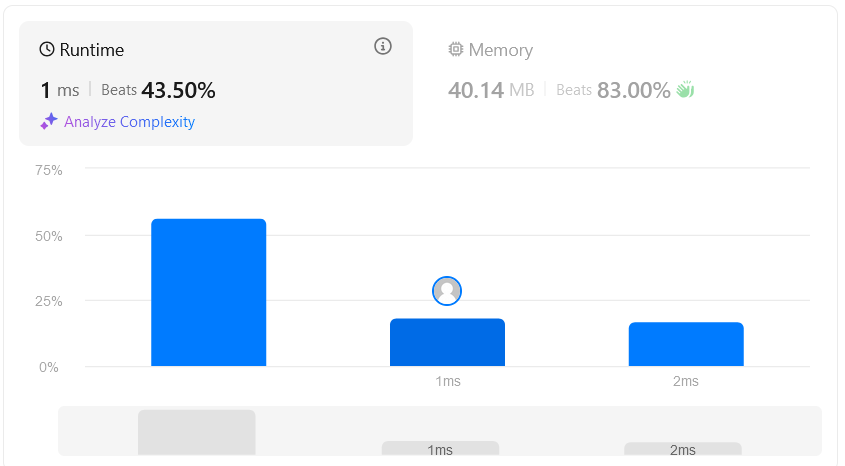