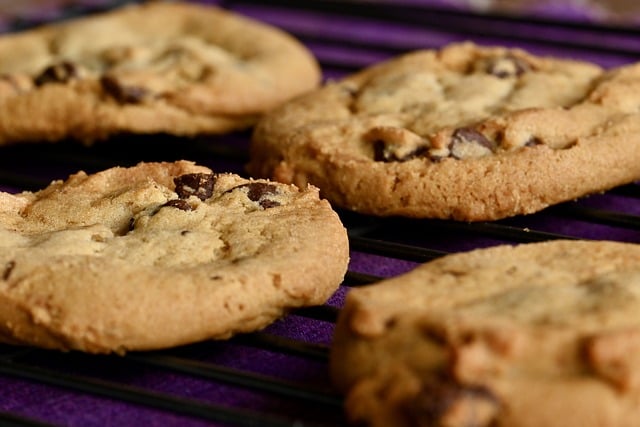
Problem Statement
This problem is from Leetcode – Assign Cookies
. The problem statement is as below:
Assume you are an awesome parent and want to give your children some cookies. But, you should give each child at most one cookie.
Each child i has a greed factor g[i], which is the minimum size of a cookie that the child will be content with; and each cookie j has a size s[j]. If s[j] >= g[i]
, we can assign the cookie j to the child i, and the child i will be content. Your goal is to maximize the number of your content children and output the maximum number.
Solution
- Sort both the given arrays.
- Iterate the arrays while checking the given condition is satisfied. If condition is satisfied then increment counter and move to the next child.
public int findContentChildren(int[] g, int[] s) {
int match = 0;
Arrays.sort(g);
Arrays.sort(s);
int i=0, j=0;
while(i<g.length && j<s.length) {
if(s[j] >= g[i]) {
match++;
// move to next child
i++;
}
// move to next cookie
j++;
}
return match;
}
You can checkout the code from Github here: Assign Cookies. See the performance of this approach below:
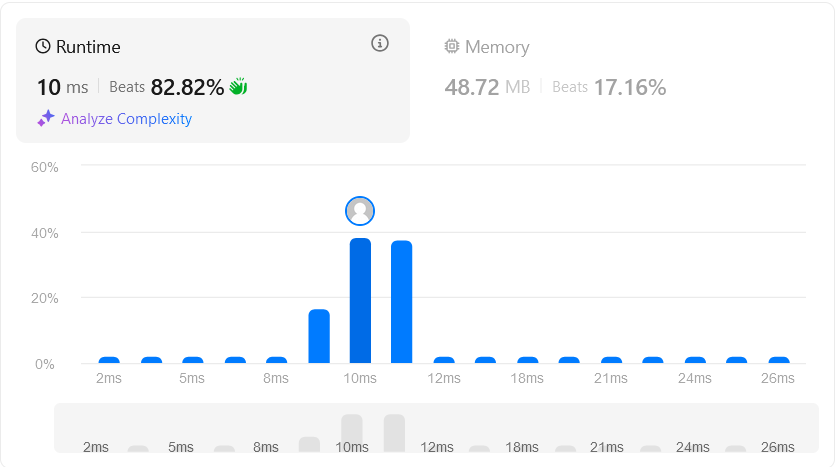