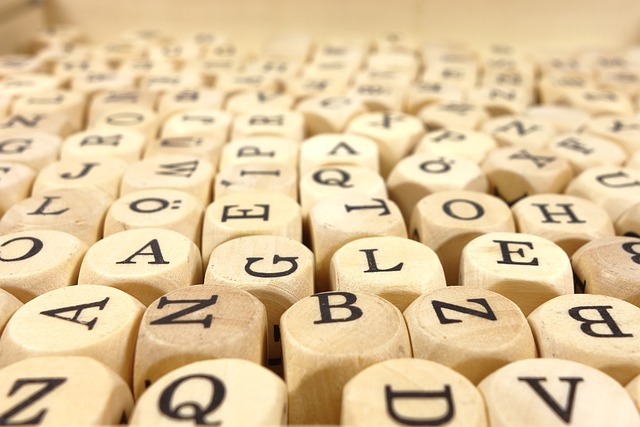
Problem Statement
This problem is from Leetcode – Number of Segments in a String
. The problem statement is given below:
Given a string s, return the number of segments in the string. A segment is defined to be a contiguous sequence of non-space characters.
Solution
Approach – 1
We can simply use a StringTokenizer
to solve this problem. See the code sample below:
public int countSegments(String s) {
// return 0 if empty string
if(s.equals("")) {
return 0;
}
StringTokenizer st = new StringTokenizer(s);
return st.countTokens();
}
You can checkout the code from Github here: Number of Segments in String – First Approach. See the performance of this approach below:
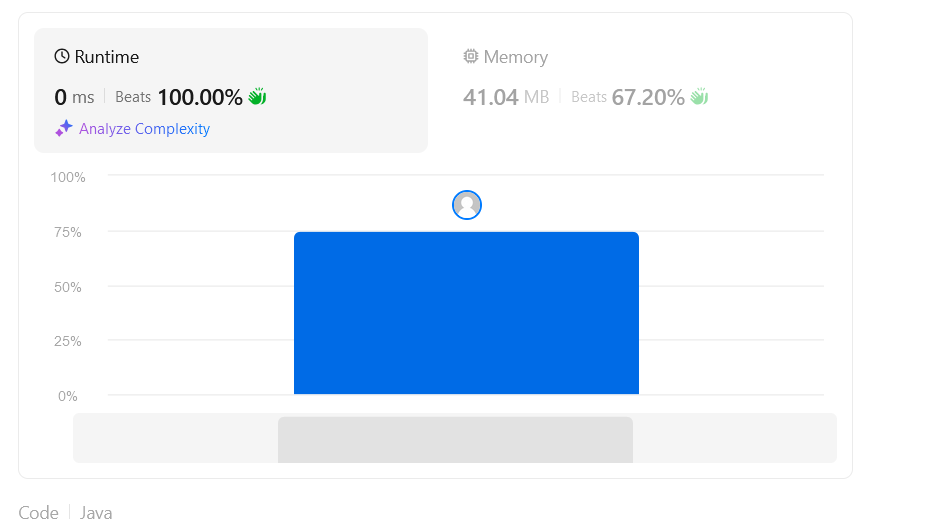
Approach – 2
We may also use good old fashioned String split()
and count the non-empty blocks. See the code sample below:
public int countSegments(String s) {
// return if string is empty
if(s.equals("")) {
return 0;
}
// initialize counter to zero - this will count no. of segments
int count = 0;
// split on " "
String[] splitarray = s.split(" ");
// in a loop count non-empty segments
for(int i=0; i<splitarray.length; i++) {
if(!splitarray[i].equals("")) {
count++;
}
}
return count;
}
You can checkout the code from Github here: Number of Segments in String – Second Approach. See the performance of this approach below:
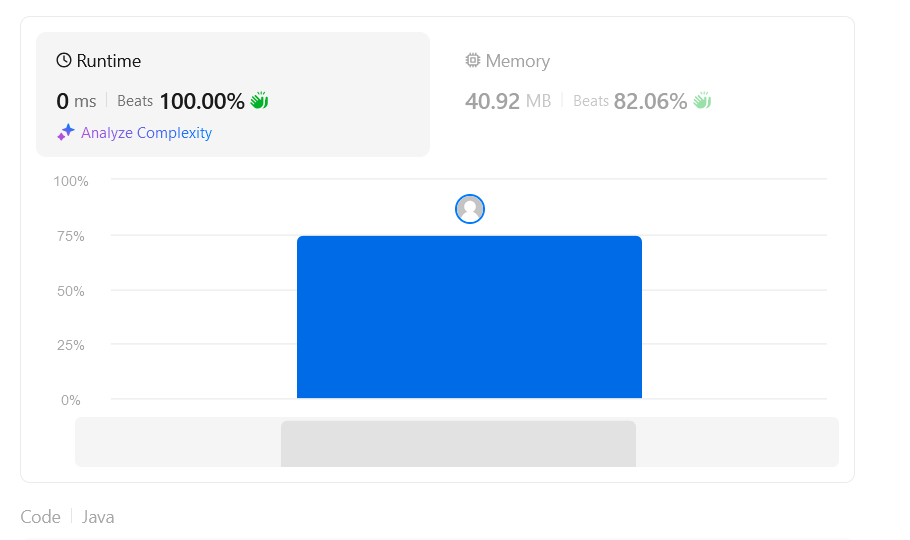