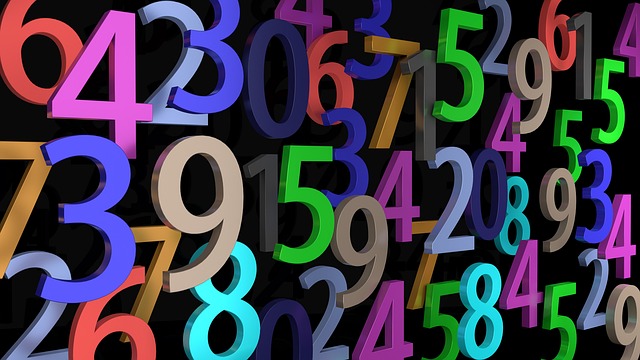
Problem Statement
This problem is from Leetcode – Reverse Integer. The problem statement is given below:
Given a signed 32-bit integer x
, return x
with its digits reversed. If reversing x
causes the value to go outside the signed 32-bit integer range [-231, 231 - 1]
, then return 0
.
Solution
Approach – 1
We need to extract individual numbers out of the given input in reverse order and append to a string. Convert this string to an integer and return. We can do this by continuously dividing input by 10 and appending the remainder to the string. See the code sample below:
public int reverse(int x) {
// if x is zero then return
if(x == 0 ) {
return 0;
}
// declare rev - this will contain our answer
int rev = 0;
// check if given integer is negative or not
boolean neg = false;
// if negative - then make it positive
if(x < 0) {
x = x * -1;
neg = true;
}
// declare empty string
String str = "";
while (x > 0) {
// append the remainder to the string
str = str + Integer.toString(x % 10) ;
// divide given integer by 10 and update it
x = x / 10;
}
// parse string back to int
try {
rev = Integer.parseInt(str.toString());
}
catch(Exception e) {
return 0;
}
// if input was negative then return negative reversed integer
if(neg) {
rev = rev * -1;
}
return rev;
}
You can checkout the code from Github here: Reverse Integer – First Approach. See the performance of this approach below:
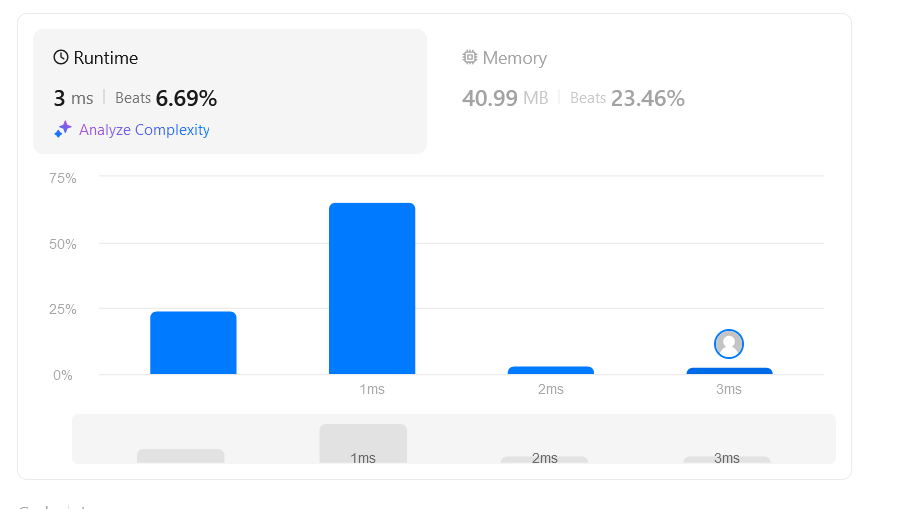
Approach – 2
We can use another simple approach. We can simply convert the given integer to string and reverse it. See the code sample below:
public int reverse(int x) {
// if x is zero then return
if(x == 0) {
return 0;
}
// declare rev - this will contain our answer
int rev = x;
// check if given integer is negative or not
boolean neg = false;
if(x < 0) {
x = x * -1;
neg = true;
}
// convert given integer to string
String str = String.valueOf(x);
// convert to StringBuilder and reverse
StringBuilder revStr = new StringBuilder(str).reverse();
// convert back to integer
try {
rev = Integer.parseInt(revStr.toString());
}
catch(Exception e) {
return 0;
}
// if input was negative then return negative reversed integer
if(neg) {
rev = rev * -1;
}
return rev;
}
You can checkout the code from Github here: Reverse Integer – Second Approach. See the performance of this approach below:
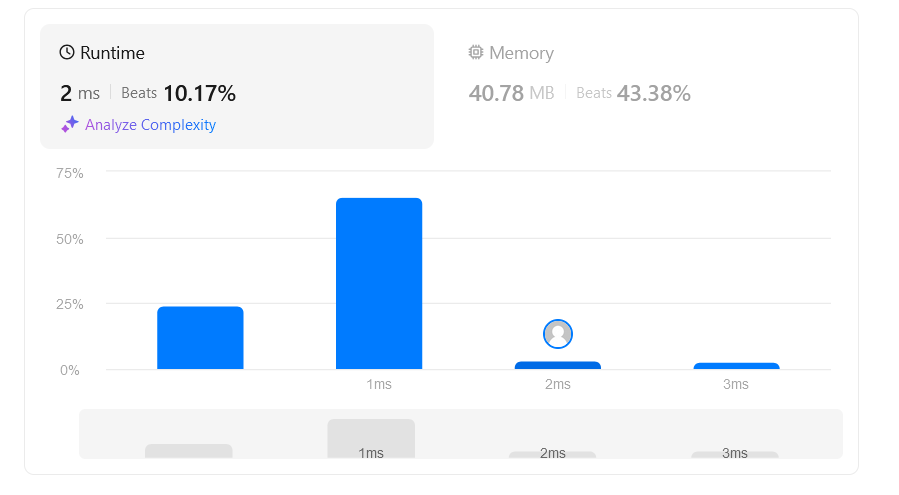
Approach – 3
Lets try another approach. We can also generate the reversed integer as we are extracting the individual numbers from Approach - 1
. See the code sample below:
public int reverse(int x) {
// if x is zero then return
if(x == 0 ) {
return 0;
}
// declare rev - this will contain our answer
long rev = 0;
// check if given integer is negative or not
boolean neg = false;
if(x < 0) {
x = x * -1;
neg = true;
}
// generate the reversed integer on the go
while (x > 0) {
rev = rev * 10 + (x % 10);
x = x / 10;
}
// if rev exceeds max limits, then return 0
if(rev > Integer.MAX_VALUE) {
return 0;
}
// if input was negative then return negative reversed integer
if(neg) {
rev = rev * -1;
}
return (int)rev;
}
You can check out the code from Github here: Reverse Integer – Third Approach. See the performance of this approach below:
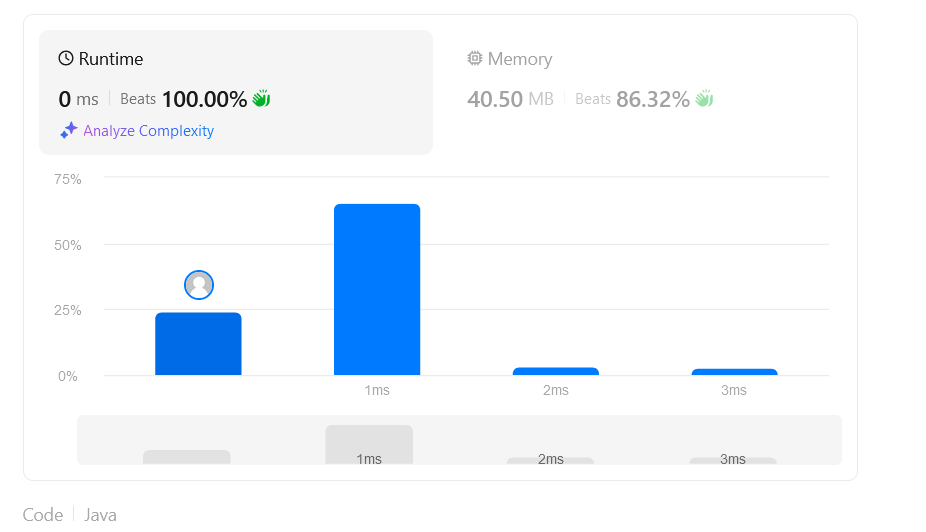